Android Login and Register with SQLite Database Tutorial
In software applications, it is mostly required to store users and app data locally. Android SDK provides several APIs for developers to save user and app data, So SQLite is one of the ways of storing data. For many applications, SQLite is the backbone of the app whether it’s used directly or via some third-party wrapper. In this tutorial, we will see how to use SQLite in our app directly.
What is SQLite?
SQLite is a lightweight database that comes with android. It is an Open-Source embedded SQL database engine. This provides a relational database management structure for storing user-defined records in the form of tables.
Key point to understand regarding SQLite :-
– SQLite is a RDBMS (Relational Database Management System)
– SQLite is written in C programming language
– SQLite is embedded within the Android operating system, so you don’t need anything external on Android to use SQLite
– To manipulate data (insert, update, delete) in SQLite database – we’ll use SQL (Structured Query Language)
Let’s Get it Working
In this tutorial we are going to learn how to use SQLite. To really understand the usage of SQLite we will create an app . The App contains simple Login form, Registration form and listing of registered user. This app shows how SQLite database operations are performed. I assume that you have created a new android project. Source Code is available on GitHub.
Step 1) Update build.gradle file.
Before you can use Material Design in your projects you need to add the following compile line to your Gradle dependencies block in your build.gradle file and rebuilt the project .
dependencies { compile 'com.android.support:appcompat-v7:23.4.0' compile 'com.android.support:design:23.4.0' compile 'com.android.support:cardview-v7:23.4.0' }
Step 2) Update strings.xml.
Add the below string values to the string.xml located in res => values => strings.xml.
<resources> <string name="app_name">Login Register</string> <string name="text_accounts">All Accounts</string> <string name="hint_name">Name</string> <string name="hint_email">Email</string> <string name="hint_password">Password</string> <string name="hint_confirm_password">Confirm Password</string> <string name="text_login">Login</string> <string name="text_register">Register</string> <string name="error_message_name">Enter Full Name</string> <string name="error_message_email">Enter Valid Email</string> <string name="error_message_age">Enter Age</string> <string name="error_message_password">Enter Password</string> <string name="success_message">Registration Successful</string> <string name="text_not_member">No account yet? Create one</string> <string name="text_already_member">Already a member? Login</string> <string name="error_email_exists">Email Already Exists</string> <string name="error_password_match">Password Does Not Matches</string> <string name="error_valid_email_password">Wrong Email or Password</string> <string name="action_settings">Settings</string> <string name="text_hello">Hello,</string> <string name="text_title">Android Tutorials Hub</string> </resources>
Step 3) Update colors.xml.
Add the below color values to the colors.xml located in res => values => colors.xml.
<?xml version="1.0" encoding="utf-8"?> <resources> <color name="colorPrimary">#51d8c7</color> <color name="colorPrimaryDark">#51d8c7</color> <color name="colorAccent">#FFFFFF</color> <color name="colorBackground">#413e4f</color> <color name="colorText">#FFFFFF</color> <color name="colorTextHint">#51d8c7</color> </resources>
Step 4) Update styles.xml.
Add the below style values to the styles.xml located in res => values => styles.xml.
<resources> <style name="AppTheme" parent="Theme.AppCompat.Light.DarkActionBar"> <item name="colorPrimary">@color/colorPrimary</item> <item name="colorPrimaryDark">@color/colorPrimaryDark</item> <item name="colorAccent">@color/colorAccent</item> <item name="android:textColor">@color/colorText</item> <item name="android:textColorHint">@color/colorText</item> <item name="colorControlNormal">@color/colorText</item> <item name="colorControlActivated">@color/colorText</item> </style> </resources>
Step 5) Add Logo Image.
Download the below logo image and add it to the drawable folder located in res => drawable.

Android Tutorials Hub Logo
Step 6) Create a User model class.
Create a new package named modal and create a User class with all getter and setter methods to maintain single contact as an object.
package com.androidtutorialshub.loginregister.model; public class User { private int id; private String name; private String email; private String password; public int getId() { return id; } public void setId(int id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getEmail() { return email; } public void setEmail(String email) { this.email = email; } public String getPassword() { return password; } public void setPassword(String password) { this.password = password; } }
Step 7) Create a DatabaseHelper class.
Create a new package named SQL and create a DatabaseHelper class. Extend this class with SQLiteOpenHelper to manage database creation and version management. I have also written some methods to manipulate data in the database.
Methods and functionality
– addUser :- add a user to the database.
– getAllUser :- fetch users data from the database.
– updateUser :- update the user in the database on the basis of the user id.
– deleteUser :- delete user from the database on the basis of the user id.
– checkUser :- check whether the user exists in the database.
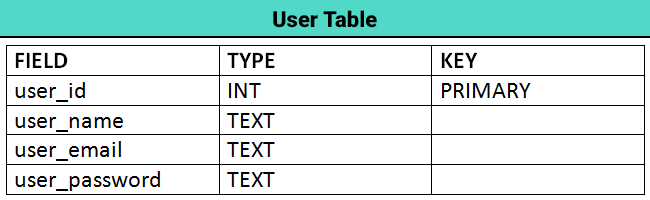
User Table Schema
package com.androidtutorialshub.loginregister.sql; import android.content.ContentValues; import android.content.Context; import android.database.Cursor; import android.database.sqlite.SQLiteDatabase; import android.database.sqlite.SQLiteOpenHelper; import com.androidtutorialshub.loginregister.model.User; import java.util.ArrayList; import java.util.List; public class DatabaseHelper extends SQLiteOpenHelper { // Database Version private static final int DATABASE_VERSION = 1; // Database Name private static final String DATABASE_NAME = "UserManager.db"; // User table name private static final String TABLE_USER = "user"; // User Table Columns names private static final String COLUMN_USER_ID = "user_id"; private static final String COLUMN_USER_NAME = "user_name"; private static final String COLUMN_USER_EMAIL = "user_email"; private static final String COLUMN_USER_PASSWORD = "user_password"; // create table sql query private String CREATE_USER_TABLE = "CREATE TABLE " + TABLE_USER + "(" + COLUMN_USER_ID + " INTEGER PRIMARY KEY AUTOINCREMENT," + COLUMN_USER_NAME + " TEXT," + COLUMN_USER_EMAIL + " TEXT," + COLUMN_USER_PASSWORD + " TEXT" + ")"; // drop table sql query private String DROP_USER_TABLE = "DROP TABLE IF EXISTS " + TABLE_USER; /** * Constructor * * @param context */ public DatabaseHelper(Context context) { super(context, DATABASE_NAME, null, DATABASE_VERSION); } @Override public void onCreate(SQLiteDatabase db) { db.execSQL(CREATE_USER_TABLE); } @Override public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) { //Drop User Table if exist db.execSQL(DROP_USER_TABLE); // Create tables again onCreate(db); } /** * This method is to create user record * * @param user */ public void addUser(User user) { SQLiteDatabase db = this.getWritableDatabase(); ContentValues values = new ContentValues(); values.put(COLUMN_USER_NAME, user.getName()); values.put(COLUMN_USER_EMAIL, user.getEmail()); values.put(COLUMN_USER_PASSWORD, user.getPassword()); // Inserting Row db.insert(TABLE_USER, null, values); db.close(); } /** * This method is to fetch all user and return the list of user records * * @return list */ public List<User> getAllUser() { // array of columns to fetch String[] columns = { COLUMN_USER_ID, COLUMN_USER_EMAIL, COLUMN_USER_NAME, COLUMN_USER_PASSWORD }; // sorting orders String sortOrder = COLUMN_USER_NAME + " ASC"; List<User> userList = new ArrayList<User>(); SQLiteDatabase db = this.getReadableDatabase(); // query the user table /** * Here query function is used to fetch records from user table this function works like we use sql query. * SQL query equivalent to this query function is * SELECT user_id,user_name,user_email,user_password FROM user ORDER BY user_name; */ Cursor cursor = db.query(TABLE_USER, //Table to query columns, //columns to return null, //columns for the WHERE clause null, //The values for the WHERE clause null, //group the rows null, //filter by row groups sortOrder); //The sort order // Traversing through all rows and adding to list if (cursor.moveToFirst()) { do { User user = new User(); user.setId(Integer.parseInt(cursor.getString(cursor.getColumnIndex(COLUMN_USER_ID)))); user.setName(cursor.getString(cursor.getColumnIndex(COLUMN_USER_NAME))); user.setEmail(cursor.getString(cursor.getColumnIndex(COLUMN_USER_EMAIL))); user.setPassword(cursor.getString(cursor.getColumnIndex(COLUMN_USER_PASSWORD))); // Adding user record to list userList.add(user); } while (cursor.moveToNext()); } cursor.close(); db.close(); // return user list return userList; } /** * This method to update user record * * @param user */ public void updateUser(User user) { SQLiteDatabase db = this.getWritableDatabase(); ContentValues values = new ContentValues(); values.put(COLUMN_USER_NAME, user.getName()); values.put(COLUMN_USER_EMAIL, user.getEmail()); values.put(COLUMN_USER_PASSWORD, user.getPassword()); // updating row db.update(TABLE_USER, values, COLUMN_USER_ID + " = ?", new String[]{String.valueOf(user.getId())}); db.close(); } /** * This method is to delete user record * * @param user */ public void deleteUser(User user) { SQLiteDatabase db = this.getWritableDatabase(); // delete user record by id db.delete(TABLE_USER, COLUMN_USER_ID + " = ?", new String[]{String.valueOf(user.getId())}); db.close(); } /** * This method to check user exist or not * * @param email * @return true/false */ public boolean checkUser(String email) { // array of columns to fetch String[] columns = { COLUMN_USER_ID }; SQLiteDatabase db = this.getReadableDatabase(); // selection criteria String selection = COLUMN_USER_EMAIL + " = ?"; // selection argument String[] selectionArgs = {email}; // query user table with condition /** * Here query function is used to fetch records from user table this function works like we use sql query. * SQL query equivalent to this query function is * SELECT user_id FROM user WHERE user_email = 'jack@androidtutorialshub.com'; */ Cursor cursor = db.query(TABLE_USER, //Table to query columns, //columns to return selection, //columns for the WHERE clause selectionArgs, //The values for the WHERE clause null, //group the rows null, //filter by row groups null); //The sort order int cursorCount = cursor.getCount(); cursor.close(); db.close(); if (cursorCount > 0) { return true; } return false; } /** * This method to check user exist or not * * @param email * @param password * @return true/false */ public boolean checkUser(String email, String password) { // array of columns to fetch String[] columns = { COLUMN_USER_ID }; SQLiteDatabase db = this.getReadableDatabase(); // selection criteria String selection = COLUMN_USER_EMAIL + " = ?" + " AND " + COLUMN_USER_PASSWORD + " = ?"; // selection arguments String[] selectionArgs = {email, password}; // query user table with conditions /** * Here query function is used to fetch records from user table this function works like we use sql query. * SQL query equivalent to this query function is * SELECT user_id FROM user WHERE user_email = 'jack@androidtutorialshub.com' AND user_password = 'qwerty'; */ Cursor cursor = db.query(TABLE_USER, //Table to query columns, //columns to return selection, //columns for the WHERE clause selectionArgs, //The values for the WHERE clause null, //group the rows null, //filter by row groups null); //The sort order int cursorCount = cursor.getCount(); cursor.close(); db.close(); if (cursorCount > 0) { return true; } return false; } }
Step 8) Create activity_login.xml.
Now create a layout file for the LoginActivity.java i.e activity_login.xml and add the below code in your layout file. The code will create a simple login form containing logo on the top, 2 input fields email and password, login button, and registration screen navigation link.
<?xml version="1.0" encoding="utf-8"?> <android.support.v4.widget.NestedScrollView xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/nestedScrollView" android:layout_width="match_parent" android:layout_height="match_parent" android:background="@color/colorBackground" android:paddingBottom="20dp" android:paddingLeft="20dp" android:paddingRight="20dp" android:paddingTop="20dp" tools:context=".activities.LoginActivity"> <android.support.v7.widget.LinearLayoutCompat android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <android.support.v7.widget.AppCompatImageView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center_horizontal" android:layout_marginTop="40dp" android:src="@drawable/logo" /> <android.support.design.widget.TextInputLayout android:id="@+id/textInputLayoutEmail" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="40dp"> <android.support.design.widget.TextInputEditText android:id="@+id/textInputEditTextEmail" android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="@string/hint_email" android:inputType="text" android:maxLines="1" android:textColor="@android:color/white" /> </android.support.design.widget.TextInputLayout> <android.support.design.widget.TextInputLayout android:id="@+id/textInputLayoutPassword" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="20dp"> <android.support.design.widget.TextInputEditText android:id="@+id/textInputEditTextPassword" android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="@string/hint_password" android:inputType="textPassword" android:maxLines="1" android:textColor="@android:color/white" /> </android.support.design.widget.TextInputLayout> <android.support.v7.widget.AppCompatButton android:id="@+id/appCompatButtonLogin" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="40dp" android:background="@color/colorTextHint" android:text="@string/text_login" /> <android.support.v7.widget.AppCompatTextView android:id="@+id/textViewLinkRegister" android:layout_width="fill_parent" android:layout_height="wrap_content" android:layout_marginTop="30dp" android:gravity="center" android:text="@string/text_not_member" android:textSize="16dp" /> </android.support.v7.widget.LinearLayoutCompat> </android.support.v4.widget.NestedScrollView>
activity_login.xml would result in a screen like this:
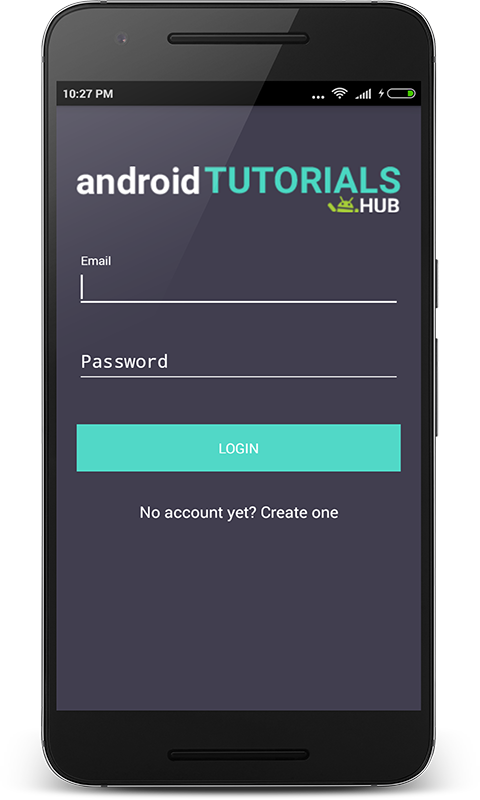
Login Screen
Step 8) Create InputValidation class.
Create a package named helpers and create a class in it named InputValidation.java and add below code in it. The code will create validation methods for the input field. Validation like empty input, valid email and etc.
package com.androidtutorialshub.loginregister.helpers; import android.app.Activity; import android.content.Context; import android.support.design.widget.TextInputEditText; import android.support.design.widget.TextInputLayout; import android.view.View; import android.view.WindowManager; import android.view.inputmethod.InputMethodManager; public class InputValidation { private Context context; /** * constructor * * @param context */ public InputValidation(Context context) { this.context = context; } /** * method to check InputEditText filled . * * @param textInputEditText * @param textInputLayout * @param message * @return */ public boolean isInputEditTextFilled(TextInputEditText textInputEditText, TextInputLayout textInputLayout, String message) { String value = textInputEditText.getText().toString().trim(); if (value.isEmpty()) { textInputLayout.setError(message); hideKeyboardFrom(textInputEditText); return false; } else { textInputLayout.setErrorEnabled(false); } return true; } /** * method to check InputEditText has valid email . * * @param textInputEditText * @param textInputLayout * @param message * @return */ public boolean isInputEditTextEmail(TextInputEditText textInputEditText, TextInputLayout textInputLayout, String message) { String value = textInputEditText.getText().toString().trim(); if (value.isEmpty() || !android.util.Patterns.EMAIL_ADDRESS.matcher(value).matches()) { textInputLayout.setError(message); hideKeyboardFrom(textInputEditText); return false; } else { textInputLayout.setErrorEnabled(false); } return true; } public boolean isInputEditTextMatches(TextInputEditText textInputEditText1, TextInputEditText textInputEditText2, TextInputLayout textInputLayout, String message) { String value1 = textInputEditText1.getText().toString().trim(); String value2 = textInputEditText2.getText().toString().trim(); if (!value1.contentEquals(value2)) { textInputLayout.setError(message); hideKeyboardFrom(textInputEditText2); return false; } else { textInputLayout.setErrorEnabled(false); } return true; } /** * method to Hide keyboard * * @param view */ private void hideKeyboardFrom(View view) { InputMethodManager imm = (InputMethodManager) context.getSystemService(Activity.INPUT_METHOD_SERVICE); imm.hideSoftInputFromWindow(view.getWindowToken(), WindowManager.LayoutParams.SOFT_INPUT_STATE_ALWAYS_HIDDEN); } }
Step 9) Create a LoginActivity class.
Now create a package named activities and create a class named LoginActivity and add below code. Here i have written the code to validate the input fields Email and Password using the InputValidation class which I described above. Also, code for navigation to registration screen on the click of registration link and to user list screen after clicking on the login button if credentials are valid.
package com.androidtutorialshub.loginregister.activities; import android.content.Intent; import android.os.Bundle; import android.support.design.widget.Snackbar; import android.support.design.widget.TextInputEditText; import android.support.design.widget.TextInputLayout; import android.support.v4.widget.NestedScrollView; import android.support.v7.app.AppCompatActivity; import android.support.v7.widget.AppCompatButton; import android.support.v7.widget.AppCompatTextView; import android.util.Log; import android.view.View; import com.androidtutorialshub.loginregister.R; import com.androidtutorialshub.loginregister.helpers.InputValidation; import com.androidtutorialshub.loginregister.sql.DatabaseHelper; public class LoginActivity extends AppCompatActivity implements View.OnClickListener { private final AppCompatActivity activity = LoginActivity.this; private NestedScrollView nestedScrollView; private TextInputLayout textInputLayoutEmail; private TextInputLayout textInputLayoutPassword; private TextInputEditText textInputEditTextEmail; private TextInputEditText textInputEditTextPassword; private AppCompatButton appCompatButtonLogin; private AppCompatTextView textViewLinkRegister; private InputValidation inputValidation; private DatabaseHelper databaseHelper; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_login); getSupportActionBar().hide(); initViews(); initListeners(); initObjects(); } /** * This method is to initialize views */ private void initViews() { nestedScrollView = (NestedScrollView) findViewById(R.id.nestedScrollView); textInputLayoutEmail = (TextInputLayout) findViewById(R.id.textInputLayoutEmail); textInputLayoutPassword = (TextInputLayout) findViewById(R.id.textInputLayoutPassword); textInputEditTextEmail = (TextInputEditText) findViewById(R.id.textInputEditTextEmail); textInputEditTextPassword = (TextInputEditText) findViewById(R.id.textInputEditTextPassword); appCompatButtonLogin = (AppCompatButton) findViewById(R.id.appCompatButtonLogin); textViewLinkRegister = (AppCompatTextView) findViewById(R.id.textViewLinkRegister); } /** * This method is to initialize listeners */ private void initListeners() { appCompatButtonLogin.setOnClickListener(this); textViewLinkRegister.setOnClickListener(this); } /** * This method is to initialize objects to be used */ private void initObjects() { databaseHelper = new DatabaseHelper(activity); inputValidation = new InputValidation(activity); } /** * This implemented method is to listen the click on view * * @param v */ @Override public void onClick(View v) { switch (v.getId()) { case R.id.appCompatButtonLogin: verifyFromSQLite(); break; case R.id.textViewLinkRegister: // Navigate to RegisterActivity Intent intentRegister = new Intent(getApplicationContext(), RegisterActivity.class); startActivity(intentRegister); break; } } /** * This method is to validate the input text fields and verify login credentials from SQLite */ private void verifyFromSQLite() { if (!inputValidation.isInputEditTextFilled(textInputEditTextEmail, textInputLayoutEmail, getString(R.string.error_message_email))) { return; } if (!inputValidation.isInputEditTextEmail(textInputEditTextEmail, textInputLayoutEmail, getString(R.string.error_message_email))) { return; } if (!inputValidation.isInputEditTextFilled(textInputEditTextPassword, textInputLayoutPassword, getString(R.string.error_message_email))) { return; } if (databaseHelper.checkUser(textInputEditTextEmail.getText().toString().trim() , textInputEditTextPassword.getText().toString().trim())) { Intent accountsIntent = new Intent(activity, UsersListActivity.class); accountsIntent.putExtra("EMAIL", textInputEditTextEmail.getText().toString().trim()); emptyInputEditText(); startActivity(accountsIntent); } else { // Snack Bar to show success message that record is wrong Snackbar.make(nestedScrollView, getString(R.string.error_valid_email_password), Snackbar.LENGTH_LONG).show(); } } /** * This method is to empty all input edit text */ private void emptyInputEditText() { textInputEditTextEmail.setText(null); textInputEditTextPassword.setText(null); } }
The Screen below shows the login form with input validation display the error message if values entered in input fields are not valid.
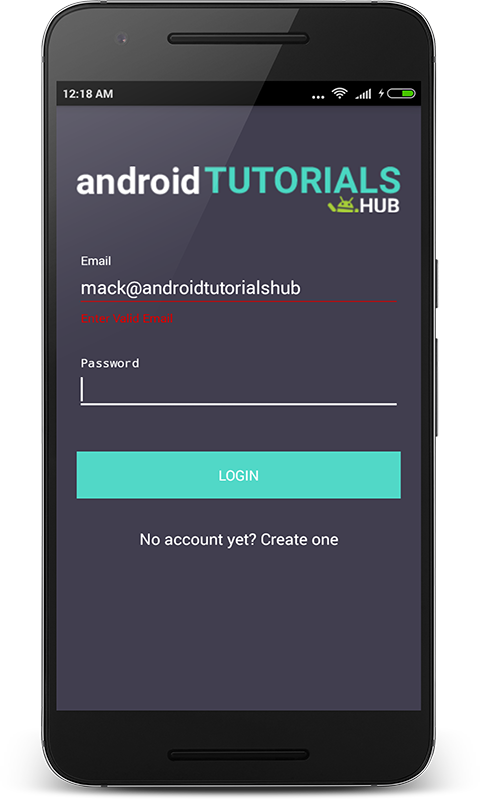
Login Screen with Input Validation
The Screen below shows the login form with input validation display the snack bar with a message if values entered in input fields are not validated from the SQLite database.
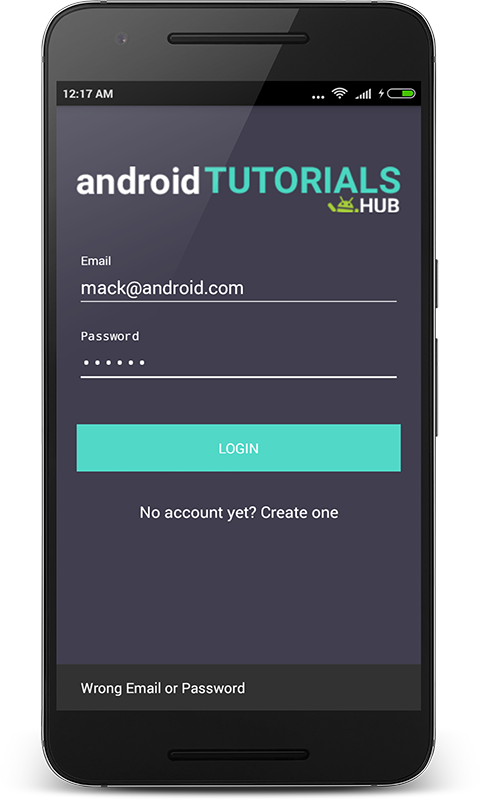
Login Screen With SQLite Validation
Step 10) Create activity_register.xml.
Now create a layout file for the RegisterActivity.java i.e activity_register.xml and add the below code in your layout file. The code will create a simple registration form containing a logo on the top, 4 input fields name, email, password, and confirm password, register button, and login screen navigation link.
<?xml version="1.0" encoding="utf-8"?> <android.support.v4.widget.NestedScrollView xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/nestedScrollView" android:layout_width="match_parent" android:layout_height="match_parent" android:background="@color/colorBackground" android:paddingBottom="20dp" android:paddingLeft="20dp" android:paddingRight="20dp" android:paddingTop="20dp" tools:context=".activities.LoginActivity"> <android.support.v7.widget.LinearLayoutCompat android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <android.support.v7.widget.AppCompatImageView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center_horizontal" android:layout_marginTop="40dp" android:src="@drawable/logo" /> <android.support.design.widget.TextInputLayout android:id="@+id/textInputLayoutName" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="40dp"> <android.support.design.widget.TextInputEditText android:id="@+id/textInputEditTextName" android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="@string/hint_name" android:inputType="text" android:maxLines="1" android:textColor="@android:color/white" /> </android.support.design.widget.TextInputLayout> <android.support.design.widget.TextInputLayout android:id="@+id/textInputLayoutEmail" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="20dp"> <android.support.design.widget.TextInputEditText android:id="@+id/textInputEditTextEmail" android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="@string/hint_email" android:inputType="text" android:maxLines="1" android:textColor="@android:color/white" /> </android.support.design.widget.TextInputLayout> <android.support.design.widget.TextInputLayout android:id="@+id/textInputLayoutPassword" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="20dp"> <android.support.design.widget.TextInputEditText android:id="@+id/textInputEditTextPassword" android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="@string/hint_password" android:inputType="textPassword" android:maxLines="1" android:textColor="@android:color/white" /> </android.support.design.widget.TextInputLayout> <android.support.design.widget.TextInputLayout android:id="@+id/textInputLayoutConfirmPassword" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="20dp"> <android.support.design.widget.TextInputEditText android:id="@+id/textInputEditTextConfirmPassword" android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="@string/hint_confirm_password" android:inputType="textPassword" android:maxLines="1" android:textColor="@android:color/white" /> </android.support.design.widget.TextInputLayout> <android.support.v7.widget.AppCompatButton android:id="@+id/appCompatButtonRegister" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="40dp" android:background="@color/colorTextHint" android:text="@string/text_register" /> <android.support.v7.widget.AppCompatTextView android:id="@+id/appCompatTextViewLoginLink" android:layout_width="fill_parent" android:layout_height="wrap_content" android:layout_marginTop="30dp" android:gravity="center" android:text="Already a member? Login" android:textSize="16dp" /> </android.support.v7.widget.LinearLayoutCompat> </android.support.v4.widget.NestedScrollView>
activity_register.xml would result in a screen like this:
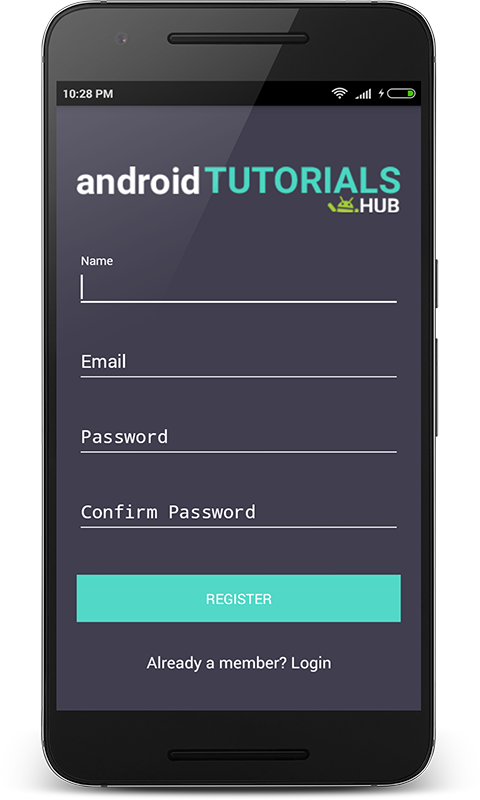
Registration Screen
Step 11) Create RegisterActivity class.
Now create a package named activities and create a class named RegisterActivity and add below code. Here I have written the code to validate the input fields Name, Email, Password, and Confirm Password using the InputValidation class which I described above. Also, code for navigation to the login screen on the click of login link and shows snackbar with success message for registration.
package com.androidtutorialshub.loginregister.activities; import android.os.Bundle; import android.support.annotation.Nullable; import android.support.design.widget.Snackbar; import android.support.design.widget.TextInputEditText; import android.support.design.widget.TextInputLayout; import android.support.v4.widget.NestedScrollView; import android.support.v7.app.AppCompatActivity; import android.support.v7.widget.AppCompatButton; import android.support.v7.widget.AppCompatTextView; import android.view.View; import com.androidtutorialshub.loginregister.R; import com.androidtutorialshub.loginregister.helpers.InputValidation; import com.androidtutorialshub.loginregister.model.User; import com.androidtutorialshub.loginregister.sql.DatabaseHelper; public class RegisterActivity extends AppCompatActivity implements View.OnClickListener { private final AppCompatActivity activity = RegisterActivity.this; private NestedScrollView nestedScrollView; private TextInputLayout textInputLayoutName; private TextInputLayout textInputLayoutEmail; private TextInputLayout textInputLayoutPassword; private TextInputLayout textInputLayoutConfirmPassword; private TextInputEditText textInputEditTextName; private TextInputEditText textInputEditTextEmail; private TextInputEditText textInputEditTextPassword; private TextInputEditText textInputEditTextConfirmPassword; private AppCompatButton appCompatButtonRegister; private AppCompatTextView appCompatTextViewLoginLink; private InputValidation inputValidation; private DatabaseHelper databaseHelper; private User user; @Override protected void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_register); getSupportActionBar().hide(); initViews(); initListeners(); initObjects(); } /** * This method is to initialize views */ private void initViews() { nestedScrollView = (NestedScrollView) findViewById(R.id.nestedScrollView); textInputLayoutName = (TextInputLayout) findViewById(R.id.textInputLayoutName); textInputLayoutEmail = (TextInputLayout) findViewById(R.id.textInputLayoutEmail); textInputLayoutPassword = (TextInputLayout) findViewById(R.id.textInputLayoutPassword); textInputLayoutConfirmPassword = (TextInputLayout) findViewById(R.id.textInputLayoutConfirmPassword); textInputEditTextName = (TextInputEditText) findViewById(R.id.textInputEditTextName); textInputEditTextEmail = (TextInputEditText) findViewById(R.id.textInputEditTextEmail); textInputEditTextPassword = (TextInputEditText) findViewById(R.id.textInputEditTextPassword); textInputEditTextConfirmPassword = (TextInputEditText) findViewById(R.id.textInputEditTextConfirmPassword); appCompatButtonRegister = (AppCompatButton) findViewById(R.id.appCompatButtonRegister); appCompatTextViewLoginLink = (AppCompatTextView) findViewById(R.id.appCompatTextViewLoginLink); } /** * This method is to initialize listeners */ private void initListeners() { appCompatButtonRegister.setOnClickListener(this); appCompatTextViewLoginLink.setOnClickListener(this); } /** * This method is to initialize objects to be used */ private void initObjects() { inputValidation = new InputValidation(activity); databaseHelper = new DatabaseHelper(activity); user = new User(); } /** * This implemented method is to listen the click on view * * @param v */ @Override public void onClick(View v) { switch (v.getId()) { case R.id.appCompatButtonRegister: postDataToSQLite(); break; case R.id.appCompatTextViewLoginLink: finish(); break; } } /** * This method is to validate the input text fields and post data to SQLite */ private void postDataToSQLite() { if (!inputValidation.isInputEditTextFilled(textInputEditTextName, textInputLayoutName, getString(R.string.error_message_name))) { return; } if (!inputValidation.isInputEditTextFilled(textInputEditTextEmail, textInputLayoutEmail, getString(R.string.error_message_email))) { return; } if (!inputValidation.isInputEditTextEmail(textInputEditTextEmail, textInputLayoutEmail, getString(R.string.error_message_email))) { return; } if (!inputValidation.isInputEditTextFilled(textInputEditTextPassword, textInputLayoutPassword, getString(R.string.error_message_password))) { return; } if (!inputValidation.isInputEditTextMatches(textInputEditTextPassword, textInputEditTextConfirmPassword, textInputLayoutConfirmPassword, getString(R.string.error_password_match))) { return; } if (!databaseHelper.checkUser(textInputEditTextEmail.getText().toString().trim())) { user.setName(textInputEditTextName.getText().toString().trim()); user.setEmail(textInputEditTextEmail.getText().toString().trim()); user.setPassword(textInputEditTextPassword.getText().toString().trim()); databaseHelper.addUser(user); // Snack Bar to show success message that record saved successfully Snackbar.make(nestedScrollView, getString(R.string.success_message), Snackbar.LENGTH_LONG).show(); emptyInputEditText(); } else { // Snack Bar to show error message that record already exists Snackbar.make(nestedScrollView, getString(R.string.error_email_exists), Snackbar.LENGTH_LONG).show(); } } /** * This method is to empty all input edit text */ private void emptyInputEditText() { textInputEditTextName.setText(null); textInputEditTextEmail.setText(null); textInputEditTextPassword.setText(null); textInputEditTextConfirmPassword.setText(null); } }
The Screen below shows the registration form with input validation display the error message if values entered in input fields are not valid.
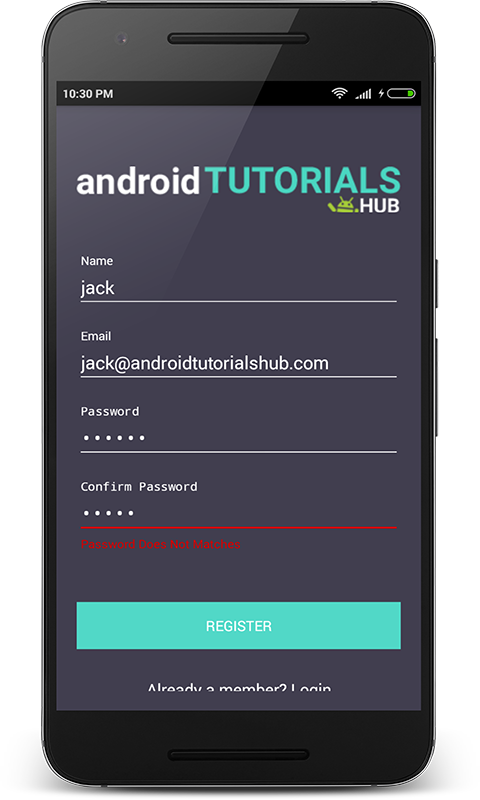
Register Screen with Input Validation
The Screen below shows the filled registration form with valid values.
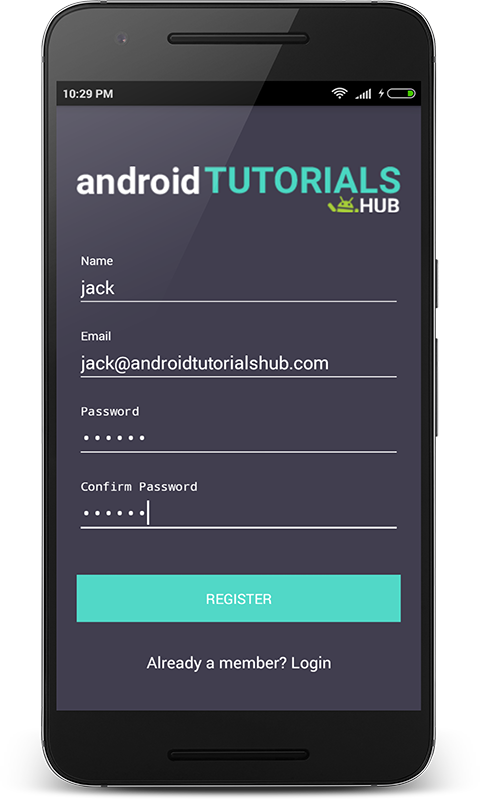
Register Screen with filled input fields
The Screen below shows the register form display the snackbar with the registration success message.
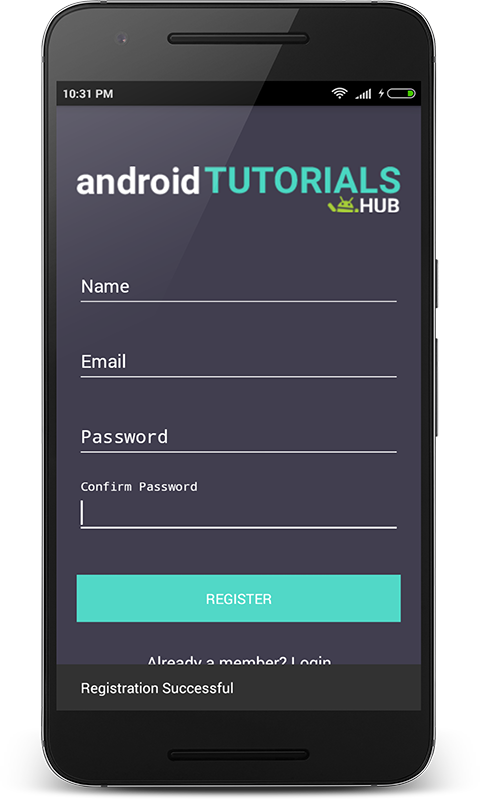
Register Screen with Registration Success Message
Step 12) Create activity_users_list.xml.
Now create a layout file for the UsersListActivity.java i.e activity_users_list.xml and add the below code in your layout file. The code will create a simple view containing two views one view i.e LinearLayout shows the welcome message with email id of the logged in user and another view i.e RecyclerView shows the list of registered users in the app.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <android.support.v7.widget.LinearLayoutCompat android:layout_width="match_parent" android:layout_height="150dp" android:background="@color/colorPrimary" android:gravity="center" android:orientation="vertical"> <android.support.v7.widget.AppCompatTextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/text_title" android:textSize="20sp" /> <android.support.v7.widget.AppCompatTextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginTop="10dp" android:text="@string/text_hello" /> <android.support.v7.widget.AppCompatTextView android:id="@+id/textViewName" android:layout_width="wrap_content" android:layout_height="wrap_content" /> </android.support.v7.widget.LinearLayoutCompat> <android.support.v7.widget.AppCompatTextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:paddingBottom="5dp" android:paddingLeft="16dp" android:paddingTop="5dp" android:text="@string/text_accounts" android:textColor="@android:color/black" /> <android.support.v7.widget.RecyclerView android:id="@+id/recyclerViewUsers" android:layout_width="match_parent" android:layout_height="match_parent" /> </LinearLayout>
activity_users_list.xml would result in a screen like this:
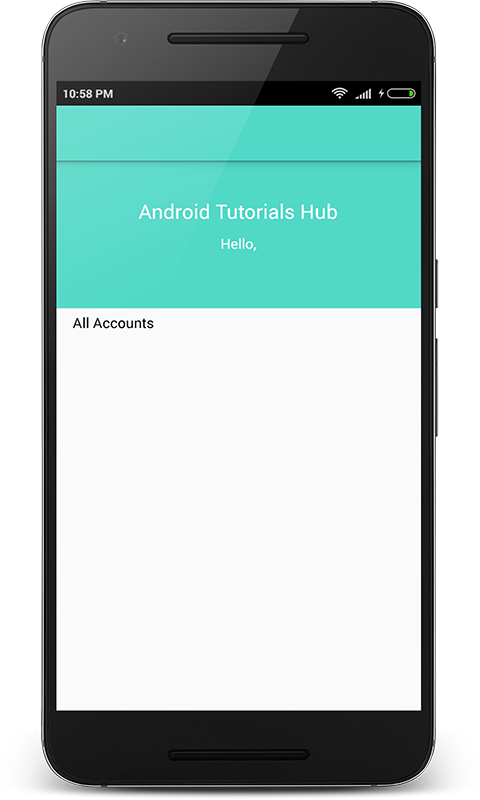
User List Screen
Step 13) Writing the Adapter Class.
Now create a package named adapters and create a class named UsersRecyclerAdapter and add below code. Here onCreateViewHolder() method inflates item_user_recycler.xml. In onBindViewHolder() method the appropriate User data (name, email, and password) set to each row.
package com.androidtutorialshub.loginregister.adapters; import android.support.v7.widget.AppCompatTextView; import android.support.v7.widget.RecyclerView; import android.util.Log; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import com.androidtutorialshub.loginregister.R; import com.androidtutorialshub.loginregister.model.User; import java.util.List; public class UsersRecyclerAdapter extends RecyclerView.Adapter<UsersRecyclerAdapter.UserViewHolder> { private List<User> listUsers; public UsersRecyclerAdapter(List<User> listUsers) { this.listUsers = listUsers; } @Override public UserViewHolder onCreateViewHolder(ViewGroup parent, int viewType) { // inflating recycler item view View itemView = LayoutInflater.from(parent.getContext()) .inflate(R.layout.item_user_recycler, parent, false); return new UserViewHolder(itemView); } @Override public void onBindViewHolder(UserViewHolder holder, int position) { holder.textViewName.setText(listUsers.get(position).getName()); holder.textViewEmail.setText(listUsers.get(position).getEmail()); holder.textViewPassword.setText(listUsers.get(position).getPassword()); } @Override public int getItemCount() { Log.v(UsersRecyclerAdapter.class.getSimpleName(),""+listUsers.size()); return listUsers.size(); } /** * ViewHolder class */ public class UserViewHolder extends RecyclerView.ViewHolder { public AppCompatTextView textViewName; public AppCompatTextView textViewEmail; public AppCompatTextView textViewPassword; public UserViewHolder(View view) { super(view); textViewName = (AppCompatTextView) view.findViewById(R.id.textViewName); textViewEmail = (AppCompatTextView) view.findViewById(R.id.textViewEmail); textViewPassword = (AppCompatTextView) view.findViewById(R.id.textViewPassword); } } }
item_user_recycler.xml
<?xml version="1.0" encoding="utf-8"?> <android.support.v7.widget.CardView xmlns:android="http://schemas.android.com/apk/res/android" xmlns:card_view="http://schemas.android.com/apk/res-auto" android:layout_width="match_parent" android:layout_height="wrap_content" card_view:cardCornerRadius="4dp"> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="vertical" android:padding="16dp"> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="horizontal"> <android.support.v7.widget.AppCompatTextView android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_weight="2" android:text="@string/hint_name" android:textColor="@color/colorTextHint" /> <android.support.v7.widget.AppCompatTextView android:id="@+id/textViewName" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_weight="1" android:text="@string/hint_name" android:textColor="@android:color/darker_gray" /> </LinearLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="5dp" android:orientation="horizontal"> <android.support.v7.widget.AppCompatTextView android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_weight="2" android:text="@string/hint_email" android:textColor="@color/colorTextHint" /> <android.support.v7.widget.AppCompatTextView android:id="@+id/textViewEmail" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_weight="1" android:text="@string/hint_email" android:textColor="@android:color/darker_gray" /> </LinearLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="5dp" android:orientation="horizontal"> <android.support.v7.widget.AppCompatTextView android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_weight="2" android:text="@string/hint_password" android:textColor="@color/colorTextHint" /> <android.support.v7.widget.AppCompatTextView android:id="@+id/textViewPassword" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_weight="1" android:text="@string/hint_password" android:textColor="@android:color/darker_gray" /> </LinearLayout> </LinearLayout> </android.support.v7.widget.CardView>
Step 14) Create UsersListActivity class.
Now create a package named activities and create a class named UsersListActivity and add below code. Here i have written the code to show the email id of the logged in user and the list of registered users.
package com.androidtutorialshub.loginregister.activities; import android.os.AsyncTask; import android.os.Bundle; import android.support.annotation.Nullable; import android.support.v7.app.AppCompatActivity; import android.support.v7.widget.AppCompatTextView; import android.support.v7.widget.DefaultItemAnimator; import android.support.v7.widget.LinearLayoutManager; import android.support.v7.widget.RecyclerView; import com.androidtutorialshub.loginregister.R; import com.androidtutorialshub.loginregister.adapters.UsersRecyclerAdapter; import com.androidtutorialshub.loginregister.model.User; import com.androidtutorialshub.loginregister.sql.DatabaseHelper; import java.util.ArrayList; import java.util.List; public class UsersListActivity extends AppCompatActivity { private AppCompatActivity activity = UsersListActivity.this; private AppCompatTextView textViewName; private RecyclerView recyclerViewUsers; private List<User> listUsers; private UsersRecyclerAdapter usersRecyclerAdapter; private DatabaseHelper databaseHelper; @Override protected void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_users_list); getSupportActionBar().setTitle(""); initViews(); initObjects(); } /** * This method is to initialize views */ private void initViews() { textViewName = (AppCompatTextView) findViewById(R.id.textViewName); recyclerViewUsers = (RecyclerView) findViewById(R.id.recyclerViewUsers); } /** * This method is to initialize objects to be used */ private void initObjects() { listUsers = new ArrayList<>(); usersRecyclerAdapter = new UsersRecyclerAdapter(listUsers); RecyclerView.LayoutManager mLayoutManager = new LinearLayoutManager(getApplicationContext()); recyclerViewUsers.setLayoutManager(mLayoutManager); recyclerViewUsers.setItemAnimator(new DefaultItemAnimator()); recyclerViewUsers.setHasFixedSize(true); recyclerViewUsers.setAdapter(usersRecyclerAdapter); databaseHelper = new DatabaseHelper(activity); String emailFromIntent = getIntent().getStringExtra("EMAIL"); textViewName.setText(emailFromIntent); getDataFromSQLite(); } /** * This method is to fetch all user records from SQLite */ private void getDataFromSQLite() { // AsyncTask is used that SQLite operation not blocks the UI Thread. new AsyncTask<Void, Void, Void>() { @Override protected Void doInBackground(Void... params) { listUsers.clear(); listUsers.addAll(databaseHelper.getAllUser()); return null; } @Override protected void onPostExecute(Void aVoid) { super.onPostExecute(aVoid); usersRecyclerAdapter.notifyDataSetChanged(); } }.execute(); } }
The Screen below after user login shows the brand name, email id of logged in user, and registered users list.
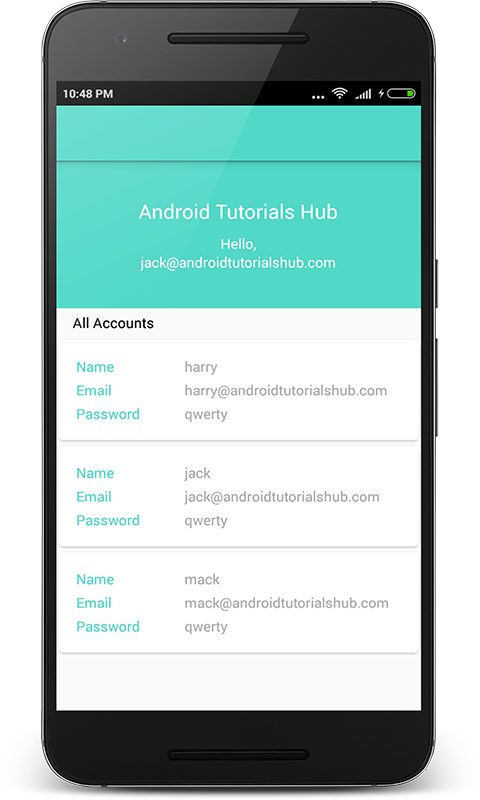
User List Screen After Login
Step 15) Update AndroidManifest.xml.
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.androidtutorialshub.loginregister"> <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:supportsRtl="true" android:theme="@style/AppTheme"> <activity android:name=".activities.LoginActivity" android:screenOrientation="portrait"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> <activity android:name=".activities.RegisterActivity" android:screenOrientation="portrait" /> <activity android:name=".activities.UsersListActivity" android:screenOrientation="portrait" /> </application> </manifest>
To learn this tutorial using KOTLIN Language Click here.
Please feel free to comment as well as ask questions. And, yeah! If this post helps you please do share!
Enjoy Coding and Share Knowledge