How to Get Hardware and Software Information in Android
We have seen many apps on play store which provides the hardware and software information. In Android one can easily fetch the information programmatically using Build class. Build class in android provides the basic information like serial number, android SDK, brand, model no, manufacture, version code and etc. There are many other information you can get from a android device but here we will focus on some of the android properties.
Let’s Get it Working
In this tutorial we are going to learn how to get hardware and software information in android. To really understand how to get hardware and software information we will create an app. This app will contains the a screen which will contain a text view to show hardware and software information and a button on which clicks hardware and software information is fetched.
Step 1) Creating New Project
Create a new project in Android Studio from File ⇒ New Project Fill the required information like project name and package name of the project. When it prompts you to select the default activity, select Empty Activity and proceed.
Step 2) Update strings.xml
Add the below string values to the strings.xml located in res ⇒ values ⇒ strings.xml.
<resources> <string name="app_name">System Information</string> <string name="get_information">Get Information</string> <string name="hard_soft_info">Hardware and Software Information</string> <string name="serial">SERIAL: </string> <string name="model">MODEL: </string> <string name="id">ID: </string> <string name="manufacturer">MANUFACTURER: </string> <string name="brand">BRAND: </string> <string name="type">TYPE: </string> <string name="user">USER: </string> <string name="base">BASE: </string> <string name="incremental">INCREMENTAL: </string> <string name="sdk">SDK: </string> <string name="board">BOARD: </string> <string name="host">HOST: </string> <string name="fingerprint">FINGERPRINT: </string> <string name="versioncode">VERSION CODE: </string> </resources>
Step 3) Create activity_main.xml
Now create a layout file for the MainActivity.java i.e activity_main.xml and add the below code in your layout file. The code will create a simple UI a scroll able text view which show the hardware and software information and a button to fetch the information.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="center_horizontal" android:orientation="vertical" android:padding="16dp" tools:context="com.androidtutorialshub.systeminformation.MainActivity"> <!-- Heading --> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/hard_soft_info" android:textSize="20sp" android:textStyle="bold" /> <!-- Scrollable information text --> <ScrollView android:layout_width="match_parent" android:layout_height="match_parent" android:layout_alignParentTop="true" android:layout_marginBottom="16dp" android:layout_marginTop="16dp" android:layout_weight="1"> <TextView android:id="@+id/textViewSetInformation" android:layout_width="wrap_content" android:layout_height="wrap_content" /> </ScrollView> <!-- To fetch information --> <Button android:id="@+id/buttonGetInformation" android:layout_width="match_parent" android:layout_height="wrap_content" android:background="@color/colorPrimary" android:text="@string/get_information" android:textColor="@android:color/white" /> </LinearLayout>
activity_main.xml would result a screen like this:
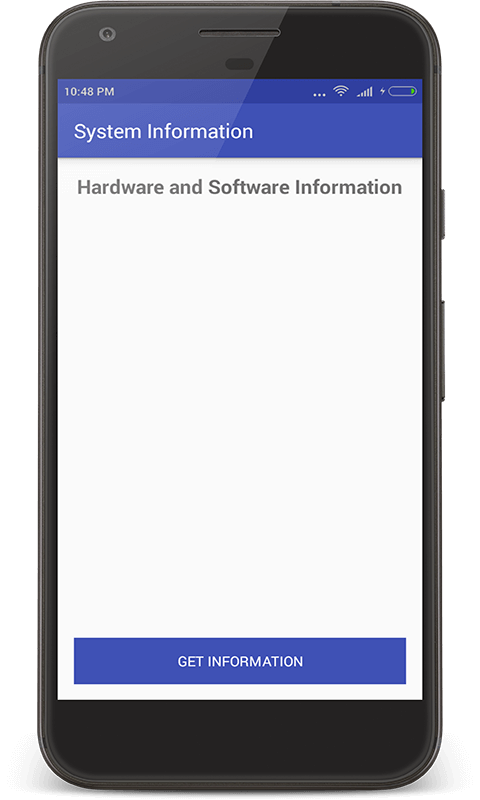
Main Screen
Step 4) Create MainActivity class
Create a class named MainActivity and add below code. Here i have written the code to fetch android hardware and software information and display it to the textview.
package com.androidtutorialshub.systeminformation; import android.os.Build; import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.view.View; import android.widget.Button; import android.widget.TextView; public class MainActivity extends AppCompatActivity { // text view to display information private TextView textViewSetInformation; // button to get information private Button buttonGetInformation; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); //initializing the views initViews(); // initializing the listeners initListeners(); } /** * method to initialize the views */ private void initViews() { textViewSetInformation = (TextView) findViewById(R.id.textViewSetInformation); buttonGetInformation = (Button) findViewById(R.id.buttonGetInformation); } /** * method to initialize the listeners */ private void initListeners() { buttonGetInformation.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { // Get hardware and software information String information = getHardwareAndSoftwareInfo(); // set information to text view textViewSetInformation.setText(information); } }); } /** * method to fetch hardware and software information * * @return information */ private String getHardwareAndSoftwareInfo() { return getString(R.string.serial) + " " + Build.SERIAL + "\n" + getString(R.string.model) + " " + Build.MODEL + "\n" + getString(R.string.id) + " " + Build.ID + "\n" + getString(R.string.manufacturer) + " " + Build.MANUFACTURER + "\n" + getString(R.string.brand) + " " + Build.BRAND + "\n" + getString(R.string.type) + " " + Build.TYPE + "\n" + getString(R.string.user) + " " + Build.USER + "\n" + getString(R.string.base) + " " + Build.VERSION_CODES.BASE + "\n" + getString(R.string.incremental) + " " + Build.VERSION.INCREMENTAL + "\n" + getString(R.string.sdk) + " " + Build.VERSION.SDK + "\n" + getString(R.string.board) + " " + Build.BOARD + "\n" + getString(R.string.host) + " " + Build.HOST + "\n" + getString(R.string.fingerprint) + " " + Build.FINGERPRINT + "\n" + getString(R.string.versioncode) + " " + Build.VERSION.RELEASE; } }
Step 5) Run App
Register the above created MainActivity in AndroidManifest.xml as a main activity and run the app by clicking run button on Android Studio and you will see the UI mentioned above, click on “Get Information” button to display the hardware and software information of your android device.
Screen when app launches
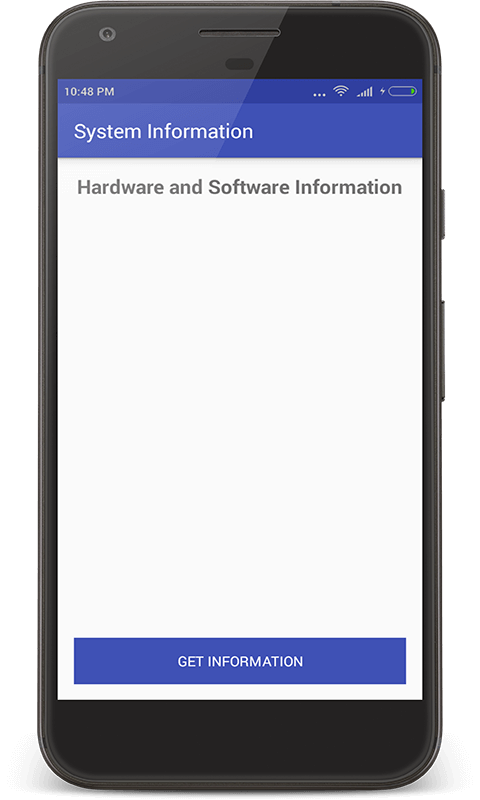
Main Screen
Screen shows android device infromation when “Get Information” Button Clicked
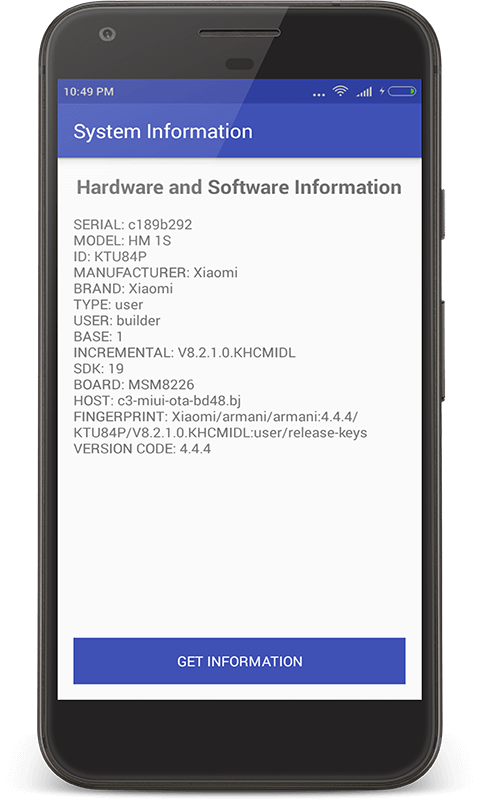
Main Screen shows information
Please feel free to comment as well as ask questions. And, yeah! If this post helps you please do share!
Enjoy Coding and Share Knowledge