Animated Gradient Background in Android
Adding Animated Gradient Background in your app is really easy by using xml and java code. Animated moving gradients background between gradients as animation makes your android app really awesome. You might have seen this type of background color animation in Instagram app’s Login Screen. Instagram app keeps on transforming background from one gradient to another very beautifully. So this can be achieved very easily by transforming gradients background using animation list.
Let’s Get it Working
In this tutorial, we are going to learn how to create a moving gradient background. To really understand the creation of animated gradient background we will create an app. This app will contain the login screen and animated background the same as you might have seen in the login screen of Instagram.
Step 1) Creating New Project
Create a new project in Android Studio from File ⇒ New Project Fill the required information like project name and package name of the project. When it prompts you to select the default activity, select Empty Activity and proceed.
Step 2) Defining strings,colors and styles
2.1 Add the below string values to the strings.xml located in res ⇒ values ⇒ strings.xml.
<resources> <string name="app_name">Animated Gradient Background</string> <string name="text_logo">Android Tutorials Hub</string> <string name="text_hint_email">Email</string> <string name="text_hint_password">Password</string> <string name="text_login">Login</string> </resources>
2.2 Add the below color values to the colors.xml located in res ⇒ values ⇒ colors.xml.
<?xml version="1.0" encoding="utf-8"?> <resources> <color name="colorPrimary">#3F51B5</color> <color name="colorPrimaryDark">#303F9F</color> <color name="colorAccent">#FFFFFF</color> <!-- 1st Gradient color --> <color name="colorPurple_A400">#D500F9</color> <color name="colorPurple_900">#4A148C</color> <!-- ./ 1st Gradient color --> <!-- 2nd Gradient color --> <color name="colorAmber_A400">#FFC400</color> <color name="colorAmber_900">#FF6F00</color> <!-- ./ 2nd Gradient color --> <!-- 3rd Gradient color --> <color name="colorGreen_A400">#00E676</color> <color name="colorGreen_900">#1B5E20</color> <!-- ./ 3rd Gradient color --> <!-- 4th Gradient color --> <color name="colorRed_A400">#FF1744</color> <color name="colorRed_900">#B71C1C</color> <!-- ./ 4th Gradient color --> </resources>
2.3 Add the below style values to the styles.xml located in res ⇒ values ⇒ styles.xml.
<resources> <!-- Base application theme. --> <style name="AppTheme" parent="Theme.AppCompat.Light.DarkActionBar"> <!-- Customize your theme here. --> <item name="colorPrimary">@color/colorPrimary</item> <item name="colorPrimaryDark">@color/colorPrimaryDark</item> <item name="colorAccent">@color/colorAccent</item> <item name="android:windowTranslucentStatus">true</item> </style> </resources>
Step 3) Adding gradient drawable
Before creating layout we have to write custom gradient drawable for the layout’s background. We will create 4 drawable resource files in a drawable folder by right click on drawable ⇒ New ⇒ Drawable resource file name is the same as the name of the following drawable XML files.
3.1 drawable_purple_gradient.xml
Add the below code to the drawable_purple_gradient.xml. This file will generate a purple color gradient background.
<?xml version="1.0" encoding="utf-8"?> <shape xmlns:android="http://schemas.android.com/apk/res/android"> <gradient android:angle="90" android:endColor="@color/colorPurple_A400" android:startColor="@color/colorPurple_900" /> </shape>
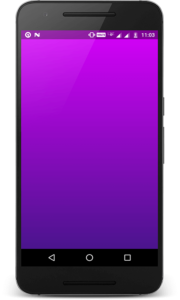
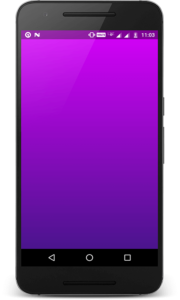
Purple Gradient Drawable
3.2 drawable_amber_gradient.xml
Add the below code to the drawable_amber_gradient.xml. This file will generate an amber color gradient background.
<?xml version="1.0" encoding="utf-8"?> <shape xmlns:android="http://schemas.android.com/apk/res/android"> <gradient android:angle="135" android:endColor="@color/colorAmber_A400" android:startColor="@color/colorAmber_900" /> </shape>
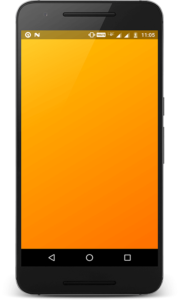
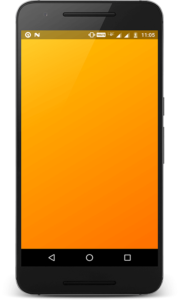
Amber Gradient Drawable
3.3 drawable_green_gradient.xml
Add the below code to the drawable_green_gradient.xml. This file will generate a green color gradient background.
<?xml version="1.0" encoding="utf-8"?> <shape xmlns:android="http://schemas.android.com/apk/res/android"> <gradient android:angle="0" android:endColor="@color/colorGreen_A400" android:startColor="@color/colorGreen_900" /> </shape>
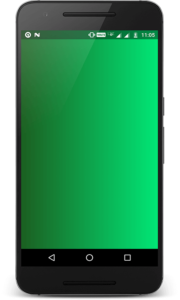
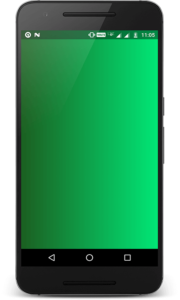
Green Gradient Drawable
3.4 drawable_red_gradient.xml
Add the below code to the drawable_red_gradient.xml. This file will generate a red color gradient background.
<?xml version="1.0" encoding="utf-8"?> <shape xmlns:android="http://schemas.android.com/apk/res/android"> <gradient android:angle="45" android:endColor="@color/colorRed_A400" android:startColor="@color/colorRed_900" /> </shape>
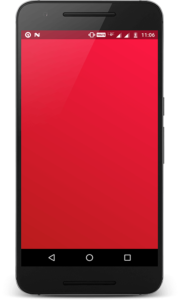
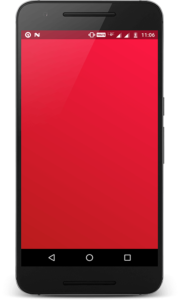
Red Gradient Drawable
Step 4) Adding gradient animation list
Again Create new drawable resource file by right click on drawable ⇒ New ⇒ Drawable resource file name it drawable_gradient_animation_list.xml and add the below code to it. This file will create an animation list for gradient drawables.
<?xml version="1.0" encoding="utf-8"?> <animation-list xmlns:android="http://schemas.android.com/apk/res/android"> <item android:drawable="@drawable/drawable_purple_gradient" android:duration="6000" /> <item android:drawable="@drawable/drawable_amber_gradient" android:duration="6000" /> <item android:drawable="@drawable/drawable_green_gradient" android:duration="6000" /> <item android:drawable="@drawable/drawable_red_gradient" android:duration="6000" /> </animation-list>
Step 5) Create activity_main.xml
Now create a layout file for the MainActivity.java i.e activity_main.xml and add the below code in your layout file. The code will create a simple login form containing logo on the top, 2 input fields email and password, login button and gradient at the background.
<?xml version="1.0" encoding="utf-8"?> <android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/constraintLayout" android:layout_width="match_parent" android:layout_height="match_parent" android:background="@drawable/drawable_gradient_animation_list" tools:context="com.androidtutorialshub.animatedgradientbackground.MainActivity"> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="vertical" android:padding="16dp" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintLeft_toLeftOf="parent" app:layout_constraintRight_toRightOf="parent" app:layout_constraintTop_toTopOf="parent"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center" android:text="@string/text_logo" android:textColor="@color/colorAccent" android:textSize="30sp" android:textStyle="bold" /> <EditText android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="50dp" android:backgroundTint="@color/colorAccent" android:hint="@string/text_hint_email" android:inputType="textEmailAddress" android:textColor="@color/colorAccent" android:textColorHint="@color/colorAccent" /> <EditText android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="10dp" android:backgroundTint="@color/colorAccent" android:fontFamily="sans-serif" android:hint="@string/text_hint_password" android:inputType="textPassword" android:textColor="@color/colorAccent" android:textColorHint="@color/colorAccent" /> <Button android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="30dp" android:background="@color/colorAccent" android:text="@string/text_login" android:textColor="@color/colorPrimary" /> </LinearLayout> </android.support.constraint.ConstraintLayout>
activity_main.xml would result a screen like this:
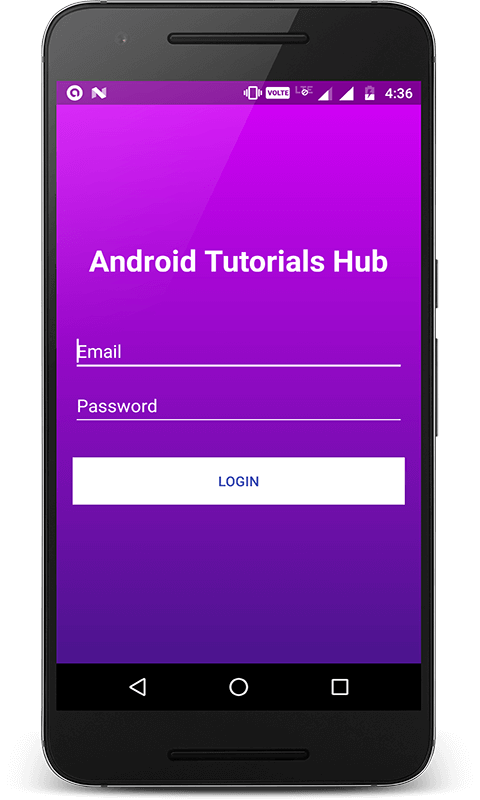
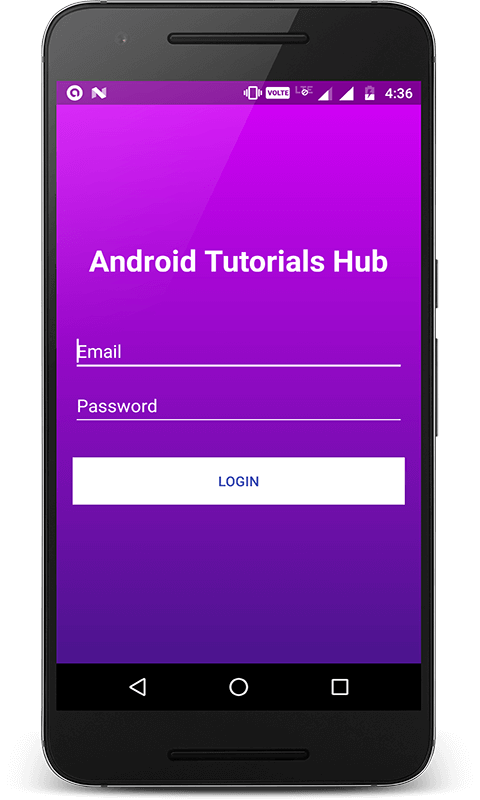
Main Screen
Step 6) Create MainActivity class
Create a class named MainActivity and add the below code. Here I have written the code to animate the background of constraint layout which I have described above in activity_main.xml.
package com.androidtutorialshub.animatedgradientbackground; import android.graphics.drawable.AnimationDrawable; import android.support.constraint.ConstraintLayout; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; public class MainActivity extends AppCompatActivity { private ConstraintLayout constraintLayout; private AnimationDrawable animationDrawable; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); getSupportActionBar().hide(); // init constraintLayout constraintLayout = (ConstraintLayout) findViewById(R.id.constraintLayout); // initializing animation drawable by getting background from constraint layout animationDrawable = (AnimationDrawable) constraintLayout.getBackground(); // setting enter fade animation duration to 5 seconds animationDrawable.setEnterFadeDuration(5000); // setting exit fade animation duration to 2 seconds animationDrawable.setExitFadeDuration(2000); } @Override protected void onResume() { super.onResume(); if (animationDrawable != null && !animationDrawable.isRunning()) { // start the animation animationDrawable.start(); } } @Override protected void onPause() { super.onPause(); if (animationDrawable != null && animationDrawable.isRunning()) { // stop the animation animationDrawable.stop(); } } }
Step 7) Run App
Register the above-created MainActivity in AndroidManifest.xml as the main activity and run the app by clicking the run button on Android Studio and see the beautiful background animation.
The Image Below shows the screen shots captured when background animation started.
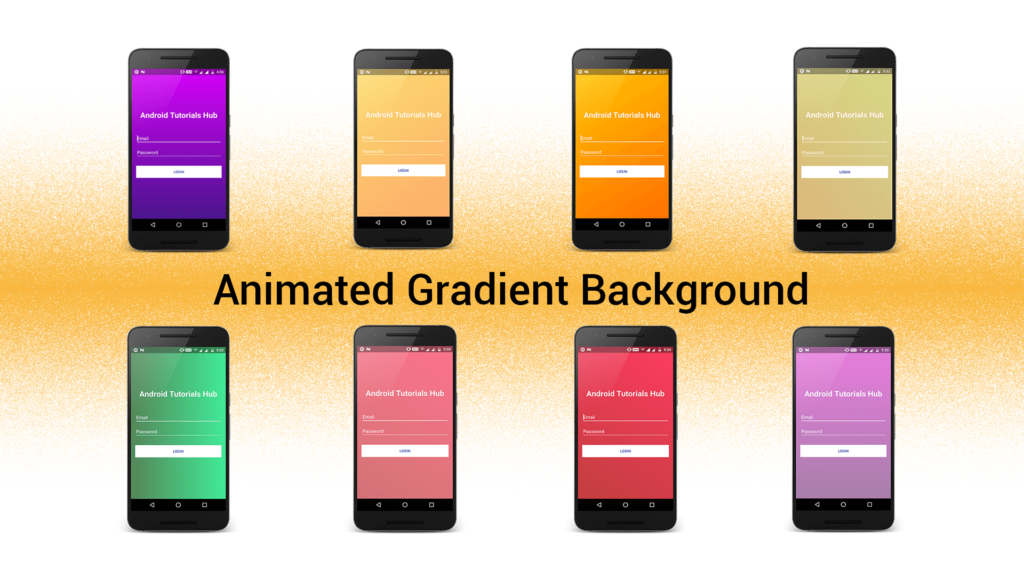
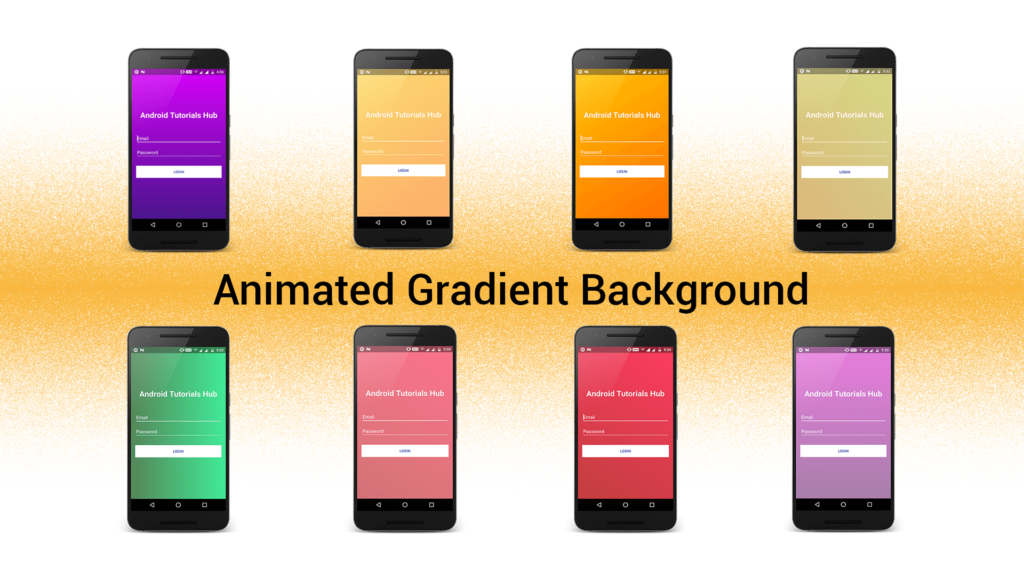
Animated Gradient Background Screens
Please feel free to comment as well as ask questions. And, yeah! If this post helps you please do share!
Enjoy Coding and Share Knowledge